ArduinoSim is a Python simulation of an Arduino Uno connected to two switches, two LEDs and a servo motor. It provides an easy way to learn how to multi-task, and was written with the following exercise in mind:
- When the top button is pressed, the green LED should blink;
- When the bottom button is pressed, the blue LED should blink;
- When both buttons are pressed, the green and blue LEDS should alternate; and
- Meanwhile, the servo should be sweeping back and forth smoothly across 180 degrees.
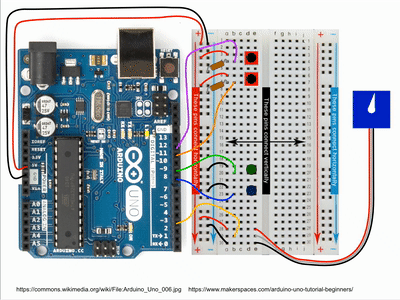
Animation showing completed exercise. (Click for larger version.)
Python
The following two files should be in your Python working folder (e.g., H:\\Python
):
Do not edit these. You will need to create a third file – you can use example.py as a template – in the same folder.
Note: The simulation is written for Python 3, and the Python module matplotlib must be installed.
When you start the Python application and command window (e.g., IDLE, or IDLEX if you have it), you will need to set the working directory:
import os os.chdir('H:\\Python')
As with the Arduino, setup()
runs once at the beginning, and then loop()
is run repeatedly.
The methods available for controlling the Arduino are:
delay(ms)
- Pause execution for ms milliseconds.
digitalRead(number)
- Returns the current value of pin number as either HIGH or LOW. Note: The pin should be set as an INPUT.
digitalWrite(number, state)
- Sets the current value of pin number as state, where state is either HIGH or LOW. Note: The pin should be set as an OUTPUT.
micros()
- Returns the number of microseconds since program started.
millis()
- Returns the number of milliseconds since program started.
pinMode(number, mode)
- Specify that pin number should be used as an input (if mode is INPUT) or as an output (if mode is OUTPUT).
Note: number is an integer in the range 0-13, corresponding to the digital pins on the Arduino.
In addition, a servo controller can be created using, e.g.:
myServo = Servo()
and this has the usual Servo methods, most importantly:
myServo.attach(number)
- Attach the servo controller to pin number.
myServo.write(angle)
- Set the servo to position angle, where 0 <= angle <= 180.